Raspberry Pi Setup
I have a Raspberry Pi v2 which I use for education (CodeClub, kids).
It ran an old Raspbian so things like GPIO support in Scratch were not available.
To make it easier for myself, I put a new SD card with Ubuntu MATE in & set it up the same way as I previously setup my LoRa gateway.
Firstly, I did an initial setup of MATE (timezone, language, computer name and initial user with encrypted home dir).
Then, I ran an upgrade, which took a while.
$ sudo su -
$ apt-get update
$ apt-get upgrade
$ reboot
After the upgrade I resized the file system via System -> Welcome -> Raspberry Pi Information screen.
Lastly, I ran rpi-update:
sudo su -
rpi-update
reboot
Minecraft Pi & GPIO
When I clicked Applications -> Games -> Minecraft Pi nothing happened.
I opened a new terminal and ran minecraft-pi manually:
$ minecraft-pi
* failed to open vchiq instance
I checked rules in /etc/udev/rules.d/. The one that caught my attention was 10-local-rpi.rules which had the following in:
SUBSYSTEM=="vchiq", GROUP="video", MODE="0660"
I checked the user who I setup initially and it wasn’t in video group:
$ groups pi
pi : pi adm cdrom sudo dip plugdev ldadmin sambashare
I added the user to the video group:
$ sudo su -
$ usermod -a -G video pi
Checked the groups again:
$ groups pi
pi : pi adm cdrom sudo dip video plugdev ldadmin sambashare
Minecraft would still not run though, because the group change takes effect only if I’d logged out and log back in again (not from the terminal, but completely).
I rebooted the pi instead.
Once back I clicked Applications -> Games -> Minecraft Pi and voilĂ , Minecraft started up.
I made a new world and kept Minecraft running.
In the terminal I checked what Python packages I have installed:
$ pip list
I noticed picraft being listed.
I checked it online. It’s an alternative library one can use for Minecraft Pi instead of mcpi.
I gave it a go:
$ python
>>> from picraft import World
>>> w = World()
>>> w.say('ahoj')
Worked as expected. Greeting ahoj appeared in Minecraft.
“Jump” Button
To conduct experiments with GPIO I made a simple circuit with a push button.
Schematic
All I need is one 1kOhm resistor, one push button and a few wires.
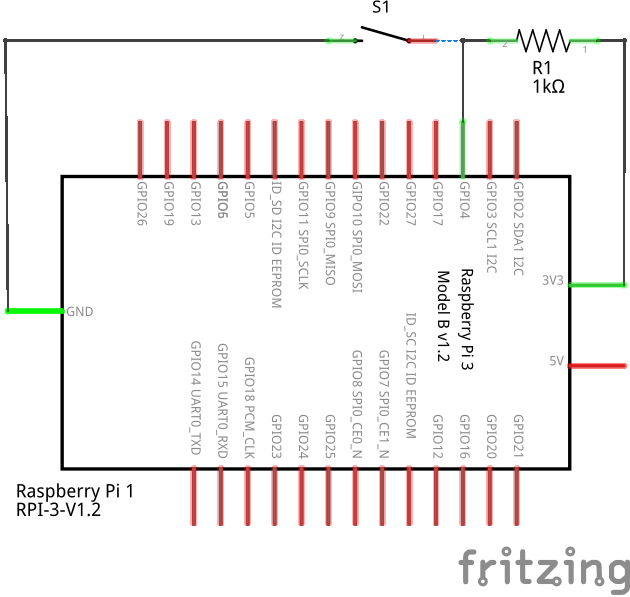
Breadboard
To know which pin is which on the pi I found pinout.xyz helpful.
Then, I wired up
- 3.3V to 1kOhm,
- 1kOhm to GPIO04 and the button,
- from the button to GND.
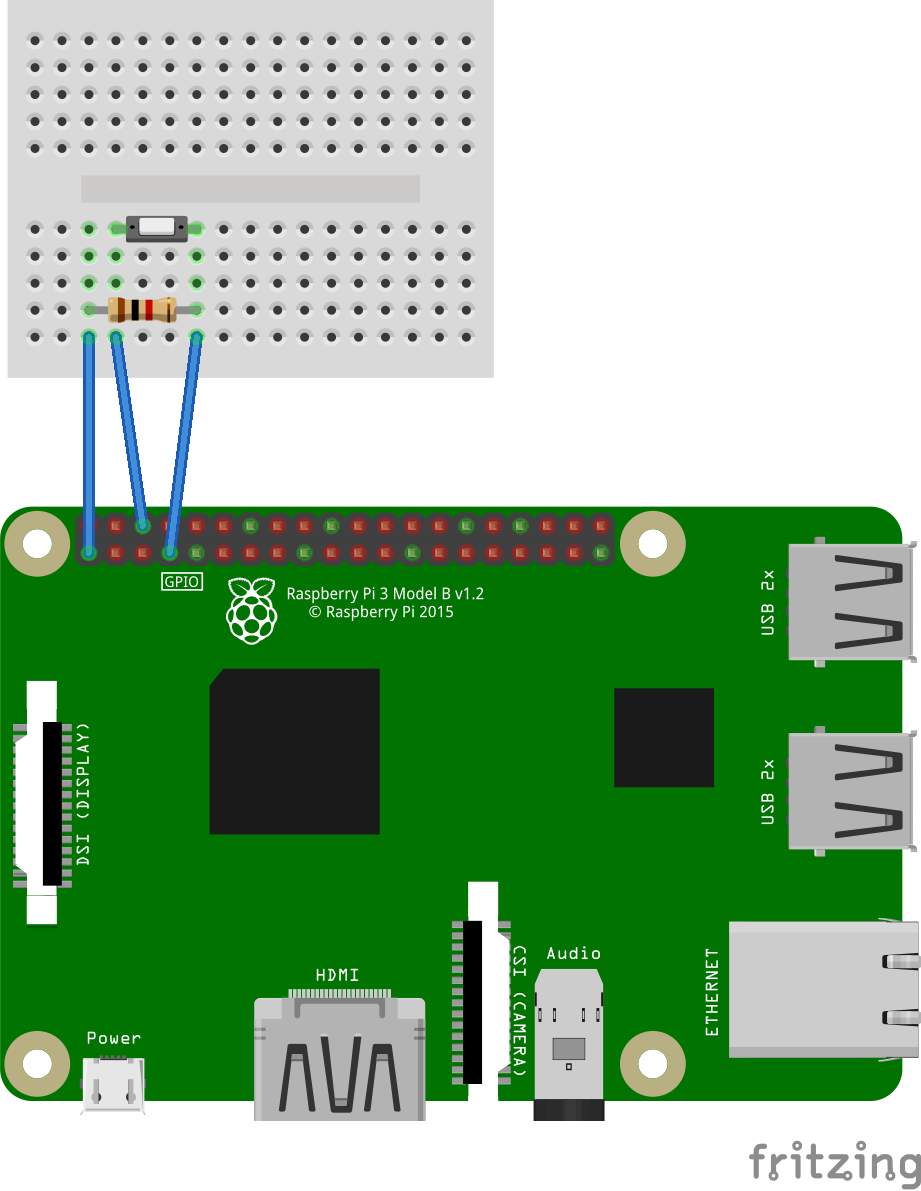
Code
I put together the following code to test the circuit.
import time
import RPi.GPIO as GPIO
from picraft import World
from picraft import Vector
# GPIO04
pin = 4
# Mode BCM eg. GPIO04 = 4
GPIO.setmode(GPIO.BCM)
# Setup GPIO04 as input
GPIO.setup(pin, GPIO.IN)
# Initialise
world = World()
# Say greeting
world.say('ahoj')
# Reset player's position to 0,0,0
world.player.pos = Vector(0,0,0)
# Initialise is button pressed
button_pressed_already = False
# Main loop
while True:
# Read button
button_pressed = GPIO.input(pin)
# When button gets pressed and is not pressed already
if button_pressed and not button_pressed_already:
# Say "jump"
world.say('jump')
# Read player's position
pos = world.player.pos
# Change player's Y position ie. jump up
world.player.pos += Vector(y=pos.y+10)
# Was the button pressed?
button_pressed_already = button_pressed
# Pause so one push of the button is only one jump
time.sleep(0.05)
Because GPIO is used I need to run the script using sudo:
pi@pi:~$ sudo python jump.py
Test
To test all the bits together, I had Minecraft Pi running and at the same time I ran sudo python jump.py in a terminal.
Firstly ahoj and then jump appeared in the Minecraft chat.
When I pressed the button, another jump appeared in chat and I jumped up and fell down again.
Comments
comments powered by Disqus